Download aplikasi yang sudah jadi disini.
Pada bagian terakhir tulisan yang membahas aplikasi gis codeigniter, kita akan mencoba menggunakan polyline dari googlemaps (baca : simple polyline). Bagian koordinat jalan kita akan membuat menggunakan polyline sebagai penanda jalan.
Beberapa library dari codeigniter akan kita pake, diantaranya library session dan cart. Jadi kita harus ngedit file config.php di dalam folder config.
File config.php edit bagian ini.
File model_koordinatjalan.php.
File koordinatjalanform.php.
Sehingga tampilannya kayak gini.
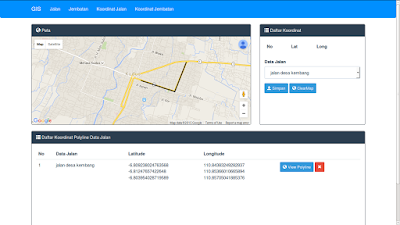
Pada bagian terakhir tulisan yang membahas aplikasi gis codeigniter, kita akan mencoba menggunakan polyline dari googlemaps (baca : simple polyline). Bagian koordinat jalan kita akan membuat menggunakan polyline sebagai penanda jalan.
Beberapa library dari codeigniter akan kita pake, diantaranya library session dan cart. Jadi kita harus ngedit file config.php di dalam folder config.
File config.php edit bagian ini.
$config['encryption_key'] = 'ISI SEMBARANG';Abis ngedit file config.php sekarang kita lanjut buat file model_koordinatjalan.php di dalam folder model.
File model_koordinatjalan.php.
<?php defined('BASEPATH') OR exit('No direct script access allowed'); class Model_koordinatjalan extends CI_Model { public function create(){ foreach ($this->cart->contents() as $koordinat) { $data = array('id_jalan' => $this->input->post('id_jalan'), 'latitude'=>$koordinat['latitude'], 'longitude'=>$koordinat['longitude']); $query = $this->db->insert('koordinatjalan', $data); } return $query; } public function getAll(){ $query = $this->db->get('koordinatjalan');//mengambil semua data koordinat jalan return $query; } public function getbyidjalan($id){ $this->db->where('id_jalan', $id); $query = $this->db->get('koordinatjalan');//mengambil semua data koordinat jalan return $query; } public function read($id){ $this->db->where('id_koordinatjalan', $id);//mengambil data koordinat jalan berdasarkan id_koordinatjalan $query = $this->db->get('koordinatjalan'); return $query; } public function update(){ $data = array('id_jalan'=>$this->input->post('id_jalan'), 'latitude'=>$this->input->post('latitude'), 'longitude'=>$this->input->post('longitude')); $this->db->where('id_koordinatjalan', $this->input->post('id_koordinatjalan'));//mengupdate berdasarkan id_koordinatjalan $query = $this->db->update('koordinatjalan', $data); return $query; } public function delete(){ $this->db->where('id_koordinatjalan', $this->input->post('id_koordinatjalan'));//menghapus berdasarkan id_koordinatjalan $query = $this->db->delete('koordinatjalan'); return $query; } public function deletebyidjalan($id){ $this->db->where('id_jalan', $id);//menghapus berdasarkan id_koordinatjalan $query = $this->db->delete('koordinatjalan'); return $query; } } /* End of file model_koordinatjalan.php */ /* Location: ./application/models/model_koordinatjalan.php */Kemudian file koordinatjalanform.php. di dalam folder view.
File koordinatjalanform.php.
<!--script google map--> <script src="https://maps.googleapis.com/maps/api/js?v=3.exp&signed_in=true"></script> <script> $(document).on('click','#clearmap',clearmap) .on('click','#simpandaftarkoordinatjalan',simpandaftarkoordinatjalan) .on('click','#hapuspolylinejalan',hapuspolylinejalan) .on('click','#viewpolylinejalan',viewpolylinejalan); var poly; var map; function initialize() { var mapOptions = { zoom: 14, // Center di kantor kecamatan jekulo center: new google.maps.LatLng(-6.806428778495534, 110.84213197231293) }; map = new google.maps.Map(document.getElementById('map-canvas'), mapOptions); var polyOptions = { strokeColor: '#000000', strokeOpacity: 1.0, strokeWeight: 3 }; poly = new google.maps.Polyline(polyOptions); poly.setMap(map); // Add a listener for the click event google.maps.event.addListener(map, 'rightclick', addLatLng); google.maps.event.addListener(map, "rightclick", function(event) { var lat = event.latLng.lat(); var lng = event.latLng.lng(); var datakoordinat = {'latitude':lat, 'longitude':lng}; $.ajax({ url : '<?php echo site_url("admin/tambahkoordinatjalan") ?>', data : datakoordinat, dataType : 'json', type : 'POST', success : function(data,status){ if (data.status!='error') { $('#daftarkoordinat').load('<?php echo current_url()."/ #daftarkoordinat > *" ?>'); }else{ alert(data.msg); } } }) //$('#latitude').val(lat); //$('#longitude').val(lng); //alert(lat +" dan "+lng); }); } /** * Handles click events on a map, and adds a new point to the Polyline. * @param {google.maps.MouseEvent} event */ function addLatLng(event) { var path = poly.getPath(); // Because path is an MVCArray, we can simply append a new coordinate // and it will automatically appear. path.push(event.latLng); // Add a new marker at the new plotted point on the polyline. var marker = new google.maps.Marker({ position: event.latLng, title: '#' + path.getLength(), map: map }); } function clearmap(e){ e.preventDefault(); $.ajax({ url : '<?php echo site_url("admin/hapusdaftarkoordinatjalan") ?>', dataType : 'json', type : 'POST', success : function(data,status){ if (data.status!='error') { $('#daftarkoordinat').load('<?php echo current_url()."/ #daftarkoordinat > *" ?>'); }else{ alert(data.msg); } } }) var mapOptions = { zoom: 14, // Center the map on Chicago, USA. center: new google.maps.LatLng(-6.805701340471898, 110.92556476593018) }; map = new google.maps.Map(document.getElementById('map-canvas'), mapOptions); var polyOptions = { strokeColor: '#000000', strokeOpacity: 1.0, strokeWeight: 3 }; poly = new google.maps.Polyline(polyOptions); poly.setMap(null); initialize(); } function simpandaftarkoordinatjalan(e){ e.preventDefault(); var datakoordinat = {'id_jalan':$('#id_jalan').val()}; console.log(datakoordinat); $.ajax({ url : '<?php echo site_url("admin/simpandaftarkoordinatjalan") ?>', dataType : 'json', data : datakoordinat, type : 'POST', success : function(data,status){ if (data.status!='error') { $('#daftarkoordinat').load('<?php echo current_url()."/ #daftarkoordinat > *" ?>'); $('#daftarkoordinatjalan').load('<?php echo current_url()."/ #daftarkoordinatjalan > *" ?>'); alert(data.msg); clearmap(e); }else{ alert(data.msg); } } }) } function hapuspolylinejalan(e){ e.preventDefault(); var datakoordinat = {'id_jalan':$(this).data('iddatajalan')}; console.log(datakoordinat); $.ajax({ url : '<?php echo site_url("admin/hapuspolylinejalan") ?>', data : datakoordinat, dataType : 'json', type : 'POST', success : function(data,status){ if (data.status!='error') { alert(data.msg); $('#daftarkoordinatjalan').load('<?php echo current_url()."/ #daftarkoordinatjalan > *" ?>'); clearmap(e); }else{ alert(data.msg); } } }) } function viewpolylinejalan(e){ e.preventDefault(); var datakoordinat = {'id_jalan':$(this).data('iddatajalan')}; console.log(datakoordinat); $.ajax({ url : '<?php echo site_url("admin/viewpolylinejalan") ?>', data : datakoordinat, dataType : 'json', type : 'POST', success : function(data,status){ if (data.status!='error') { clearmap(e); //load polyline $.each(data.msg,function(m,n){ var lat = n["latitude"]; var lng = n["longitude"]; console.log(m,n); $.each(data.datajalan,function(k,v){ createpolylinedatajalan(data.msg,v['namajalan'],lat,lng); }) return false; }) //end load polyline }else{ alert(data.msg); } } }) } function createpolylinedatajalan(datakoordinat,nama,lat,lon){ var mapOptions = { zoom: 14, //get center latlong center: new google.maps.LatLng(lat, lon), //mapTypeId: google.maps.MapTypeId.TERRAIN //end get center latlong }; var map = new google.maps.Map(document.getElementById('map-canvas'), mapOptions); var listkoordinat = []; $.each(datakoordinat,function(k,v){ listkoordinat.push(new google.maps.LatLng(parseFloat(v['latitude']), parseFloat(v['longitude']))); }) var pathKoordinat = new google.maps.Polyline({ path: listkoordinat, geodesic: true, strokeOpacity: 1.0, }); pathKoordinat.setMap(map); } google.maps.event.addDomListener(window, 'load', initialize); </script> <!--end script google map--> <div class="container"> <div class="row"> <div class="col-md-8 col-sm-8"> <div class="panel panel-primary"> <div class="panel-heading"><span class="glyphicon glyphicon-globe"></span> Peta</div> <div class="panel-body" style="height:300px;" id="map-canvas"> </div> </div> </div> <div class="col-md-4 col-sm-4"> <div class="panel panel-primary"> <div class="panel-heading"><span class="glyphicon glyphicon-list"></span> Daftar Koordinat</div> <div class="panel-body" style="min-height:300px;"> <table class="table"> <th>No</th> <th>Lat</th> <th>Long</th> <th></th> <tbody id="daftarkoordinat"> <?php if($this->cart->contents()!=null){ foreach ($this->cart->contents() as $koordinat) { if($koordinat['jenis']=='jalan'){ echo '<tr><td>'.$koordinat["id"].'</td><td>'.$koordinat["latitude"].'</td><td>'.$koordinat["longitude"].'</td></tr>'; } } } ?> </tbody> </table> <form action="#"> <div class="form-group"> <label for="datajalan">Data Jalan</label> <?php if ($itemdatajalan->num_rows()!=null) { echo '<select name="id_jalan" id="id_jalan" class="form-control">'; foreach ($itemdatajalan->result() as $datajalan) { echo "<option value='".$datajalan->id_jalan."'>".$datajalan->namajalan."</option>"; } echo '</select>'; }else{ echo anchor('admin/jalan', '<span class="glyphicon glyphicon-plus"></span> Tambah Data Jalan', 'class="btn btn-info form-control"'); } ?> </div> <div class="form-group"> <button class="btn btn-info btn-sm" id="simpandaftarkoordinatjalan"><span class="glyphicon glyphicon-save"></span> Simpan</button> <button class="btn btn-info btn-sm" id="clearmap"><span class="glyphicon glyphicon-globe"></span> ClearMap</button> </div> </form> </div> </div> </div> <div class="col-md-12 col-sm-12"> <div class="panel panel-primary"> <div class="panel-heading"><span class="glyphicon glyphicon-th-list"></span> Daftar Koordinat Polyline Data Jalan</div> <div class="panel-body" style="min-height:400px"> <table class="table"> <th>No</th> <th>Data Jalan</th> <th>Latitude</th> <th>Longitude</th> <th></th> <tbody id="daftarkoordinatjalan"> <?php if ($itemkoordinatjalan->num_rows()!=null) { $no = 1; foreach ($itemdatajalan->result() as $jalan) { echo "<tr>"; echo "<td>"; echo $no++; echo "</td>"; echo "<td>"; echo $jalan->namajalan; echo "</td>"; echo "<td>"; foreach ($itemkoordinatjalan->result() as $koordinat) { if ($koordinat->id_jalan==$jalan->id_jalan) { echo $koordinat->latitude."</br>"; } } echo "</td>"; echo "<td>"; foreach ($itemkoordinatjalan->result() as $koordinat) { if ($koordinat->id_jalan==$jalan->id_jalan) { echo $koordinat->longitude."</br>"; } } echo "</td>"; echo "<td>"; echo '<button class="btn-info btn btn-sm" id="viewpolylinejalan" data-iddatajalan="'.$jalan->id_jalan.'"><span class="glyphicon-globe glyphicon"></span> View Polyline</button> '; echo '<button class="btn-danger btn btn-sm" id="hapuspolylinejalan" data-iddatajalan="'.$jalan->id_jalan.'"><span class="glyphicon-remove glyphicon"></span></button>'; echo "</td>"; echo "</tr>"; } } ?> </tbody> </table> </div> </div> </div> </div> </div>Terakhir file admin.php di folder controller tambahi beberapa baris code.
//crud koordinat jalan () public function koordinatjalan(){ $data = array('content' => 'admin/koordinatjalanform', 'itemdatajalan'=>$this->model_jalan->getAll(), 'itemkoordinatjalan'=>$this->model_koordinatjalan->getAll()); $this->load->view('templates/template', $data, FALSE); } function tambahkoordinatjalan() { if(!$this->input->is_ajax_request()) { show_404(); }else { if($this->cart->contents()==null){ $data = array( 'id' => 1, 'qty' => 1, 'price' => 1, 'jenis' => 'jalan', 'name' => 1, 'latitude'=> $this->input->post('latitude'), 'longitude'=> $this->input->post('longitude') ); $this->cart->insert($data); $status = "success"; $msg = "<div class='alert alert-success'>Data berhasil disimpan</div>"; }else{ $urut = 0; foreach ($this->cart->contents() as $jalan) { $urut +=1; } $data = array( 'id' => $urut + 1, 'qty' => 1, 'price' => 1, 'jenis' => 'jalan', 'name' => $urut + 1, 'latitude'=> $this->input->post('latitude'), 'longitude'=> $this->input->post('longitude') ); $this->cart->insert($data); $status = "success"; $msg = "<div class='alert alert-success'>Data berhasil disimpan</div>"; } $this->output->set_content_type('application/json')->set_output(json_encode(array('status'=>$status,'msg'=>$msg))); } } function hapusdaftarkoordinatjalan(){ if (!$this->input->is_ajax_request()) { show_404(); }else{ $hapus = $this->cart->destroy(); $status = 'success'; $msg = 'data koordinat berhasil dihapus'; $this->output->set_content_type('application/json')->set_output(json_encode(array('status'=>$status,'msg'=>$msg))); } } function simpandaftarkoordinatjalan(){ if (!$this->input->is_ajax_request()) { show_404(); }else{ $this->load->library('form_validation'); $this->form_validation->set_rules('id_jalan', 'Data Jalan', 'trim|required'); if ($this->form_validation->run()==false) { $status = 'error'; $msg = validation_errors(); }else{ if ($this->model_koordinatjalan->getbyidjalan($this->input->post('id_jalan'))->num_rows()!=null) { $status = 'error'; $msg = 'polyline jalan yang bersangkutan sudah ada, hapus terlebih dahulu'; }else{ if ($this->model_koordinatjalan->create()) { $status = 'success'; $msg = 'data berhasil disimpan'; $this->cart->destroy(); }else{ $status = 'error'; $msg = 'terjadi kesalahan saat menyimpan koordinat'; } } } $this->output->set_content_type('application/json')->set_output(json_encode(array('status'=>$status,'msg'=>$msg))); } } function hapuspolylinejalan(){ if (!$this->input->is_ajax_request()) { show_404(); }else{ if ($this->model_koordinatjalan->deletebyidjalan($this->input->post('id_jalan'))) { $status = 'success'; $msg = 'data berhasil dihapus'; }else{ $status = 'error'; $msg = 'terjadi kesalahan saat menghapus data'; } $this->output->set_content_type('application/json')->set_output(json_encode(array('status'=>$status,'msg'=>$msg))); } } function viewpolylinejalan(){ if (!$this->input->is_ajax_request()) { show_404(); }else{ if ($this->model_koordinatjalan->getbyidjalan($this->input->post('id_jalan'))->num_rows()!=null){ $status = 'success'; $msg = $this->model_koordinatjalan->getbyidjalan($this->input->post('id_jalan'))->result(); $datajalan = $this->model_jalan->read($this->input->post('id_jalan'))->result(); }else{ $status = 'error'; $msg = 'data tidak ditemukan'; $datajalan = null; } $this->output->set_content_type('application/json')->set_output(json_encode(array('status'=>$status,'msg'=>$msg,'datajalan'=>$datajalan))); } } //end crud koordinat jalanKalo udah semua sekarang kita coba buka dengan mengakses http://localhost/simplegis/index.php/admin/koordinatjalan
Sehingga tampilannya kayak gini.
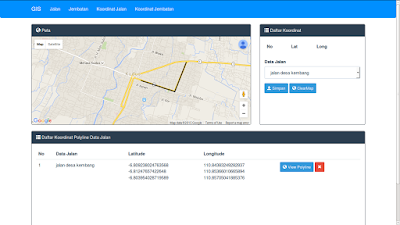
Tidak ada komentar:
Posting Komentar